API Details
IMPORTANT!
If API requests are made from a client-side URL, then that URL must be whitelisted due to cross domain restrictions.
More information is available under the API/Access Control Tab in your EmailOversight user account.
EmailOversight API Services
Available APIs
- Email Validation
- Phone Validation
- Activity Match
- Address Validation
- Email Append
- IP Email Append
- Name Append
- Address Append
- Zip Append
- Age Append
- DOB Append
- Gender Append
- Ethnicity Append
- Data Append
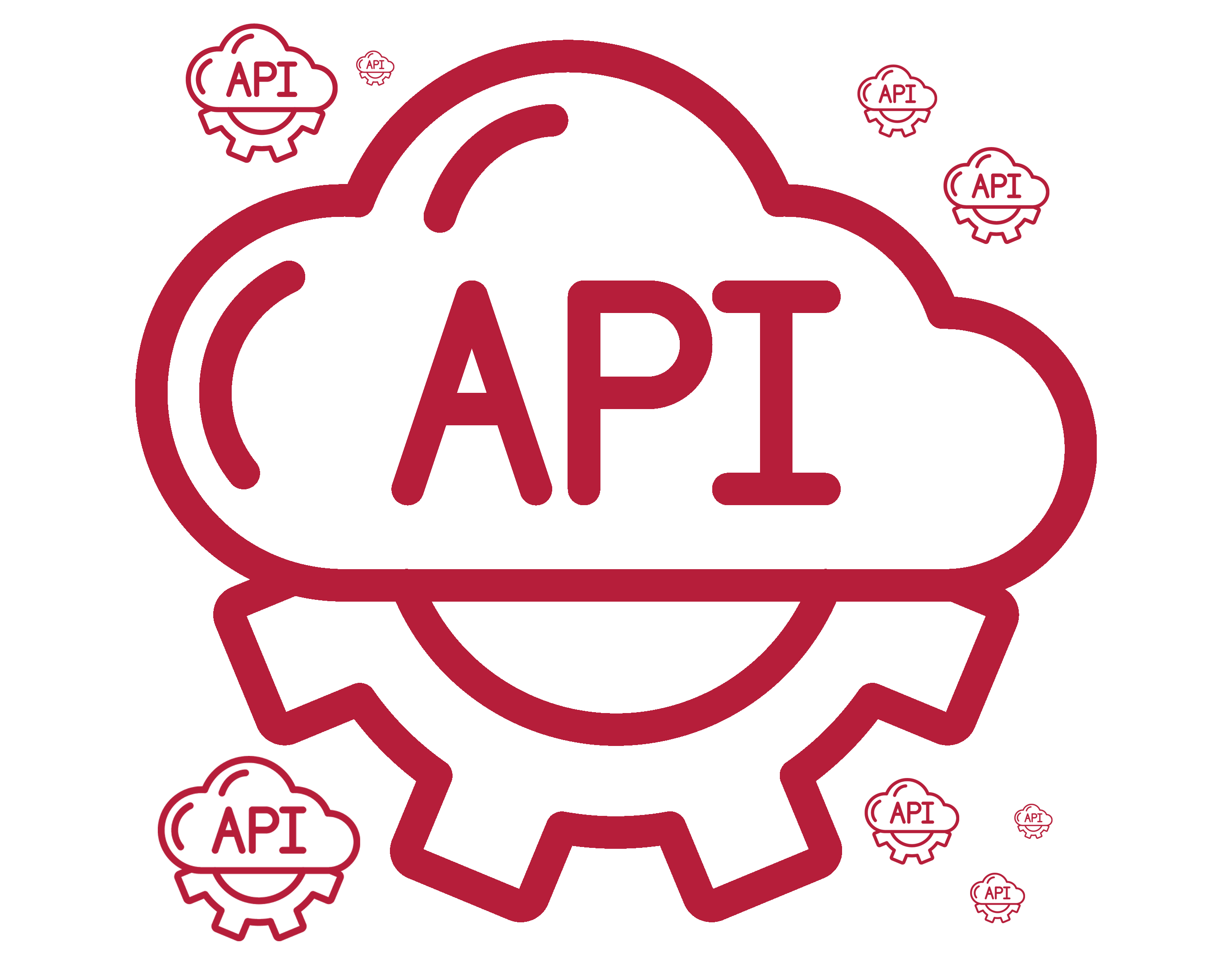
IMPORTANT NOTE:
The following details pertain to EmailOversight’s Email Validation API.
API Parameters
List IDs are located under Lists menu. List ID must be valid and belong to the user. Request will return an error if ListId is invalid.
Email:
Email address to be validated.
GET Method
string format.
GET URL Example
GET
https://api.emailoversight.com/api/emailvalidation?apitoken={API Token}&listid={ListId}&email={Email}
{API Token} = 00000xx0-00×0-00×0-00xx-x00xx000xxxx
==> https://api.emailoversight.com/api/emailvalidation?apitoken=00000xx0-00×0-00×0-00xx-x00xx000xxxx&listid={ListId}&email={Email}
POST Method
IMPORTANT!
POST URL Example
Request Header Example
Request Header
“ApiToken”:”{API Token}”
{API Token} = 00000xx0-00×0-00×0-00xx-x00xx000xxxx
==> “ApiToken”:”00000xx0-00×0-00×0-00xx-x00xx000xxxx”
Request Body Example (JSON)
Request Body {“ListId”:{ListId},”Email”:{Email}}
{ListId} = 25
{Email} = “joe@testdomain.com”
==> {“ListId”:25,”Email”:”joe@testdomain.com”}
Email Validation API Response
API Response Example (JSON)(POST Method)
API Response Format { “ListId”:{ListId}, “Email”:{Email}, “ResultId”:{ResultId}, “Result”:{Result} }
{API Token} = 00000xx0-00×0-00×0-00xx-x00xx000xxxx
{ListId} = 25
{Email} = “joe@testdomain.com”
GET Method
https://api.emailoversight.com/api/emailvalidation?apitoken=00000xx0-00×0-00×0-00xx-x00xx000xxxx&listid={ListId}&email={Email}
POST Method
https://api.emailoversight.com/api/emailvalidation
Request Header “ApiToken”:”00000xx0-00×0-00×0-00xx-x00xx000xxxx”
Request Body {“ListId”:25,”Email”:”joe@testdomain.com”}
{“ListId”:25, “Email”:”joe@testdomain.com”, “ResultId”:1, “Result”:”Verified”}
Email Validation Result Table
Code Examples
JavaScript Code Examples
GET Method
<script src="Scripts/jquery-2.1.1.js"></script>
<script>
function EmailValidationGet(apiToken, listId, email) {
$.ajaxSetup({ contentType: 'application/json; charset=utf-8' });
$.getJSON("https://api.emailoversight.com/api/emailvalidation?apitoken=" + apiToken + "&listid=" + listId + "&email=" + email, function (data) {
}).done(function (data) {
var str = JSON.stringify(data, null, 2);
//Usable Json Object
var obj = JSON.parse(str);
})
.fail(function (jqxhr, textStatus, error) {
//Available fields
console.log(jqxhr["responseJSON"]);
console.log(jqxhr["readyState"]);
console.log(jqxhr["responseText"]);
console.log(jqxhr["status"]);
console.log(jqxhr["statusText"]);
});
}
</script>
POST Method
<script src="Scripts/jquery-2.1.1.js"></script>
<script>
function EmailValidationPost(apiToken, listId, email) {
var requestJson = { 'ListId':listId, 'Email':email };
$.ajax("https://api.emailoversight.com/api/emailvalidation", {
type: "POST",
data: JSON.stringify(requestJson),
contentType: 'application/json; charset=utf-8',
dataType: 'json',
headers: GetHeaders(),
success: function (data) {
var str = JSON.stringify(data, null, 2);
//Usable Json Object
var obj = JSON.parse(str);
},
error: function (jqxhr, textStatus, error) {
//Available fields
console.log(jqxhr["responseJSON"]);
console.log(jqxhr["readyState"]);
console.log(jqxhr["responseText"]);
console.log(jqxhr["status"]);
console.log(jqxhr["statusText"]);
}
});
}
function GetHeaders(){
return { "ApiToken": "00000xx0-00x0-00x0-00xx-x00xx000xxxx" };
}
</script>
C# Code Examples
GET Method
using System.Net.Http;
using System.Net.Http.Headers;
public class EmailValidationRequest
{
public int ListId { get; set; }
public string Email { get; set; }
}
public class EmailValidationResponse
{
public int ListId { get; set; }
public string Email { get; set; }
public int ResultId { get; set; }
public string Result { get; set; }
}
static string _apiURL = "https://api.emailoversight.com/api/emailvalidation";
static string _apiToken = "00000xx0-00x0-00x0-00xx-x00xx000xxxx";
public static EmailValidationResponse ValidateEmail(EmailValidationRequest request, int timeoutInSeconds, Logger log)
{
EmailValidationResponse resps = new EmailValidationResponse();
using (var client = new HttpClient())
{
try
{
client.BaseAddress = new Uri(string.Format("{0}?apitoken={1}&listid={2}&email={3}", _apiURL, _apiToken, request.ListId, request.Email));
client.DefaultRequestHeaders.Accept.Clear();
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
client.Timeout = new TimeSpan(0, 0, 0, timeoutInSeconds);
HttpResponseMessage response = client.GetAsync("").Result;
if (response.IsSuccessStatusCode)
{
resps = response.Content.ReadAsAsync<EmailValidationResponse>().Result;
}
else
{
// Incase of an error
log.Trace(response.ReasonPhrase);
}
}
catch (Exception ex)
{
log.Trace(ex);
}
}
return resps;
}
POST Method
using System.Net.Http;
using System.Net.Http.Headers;
public class EmailValidationRequest
{
public int ListId { get; set; }
public string Email { get; set; }
}
public class EmailValidationResponse
{
public int ListId { get; set; }
public string Email { get; set; }
public int ResultId { get; set; }
public string Result { get; set; }
}
static string _apiURL = "https://api.emailoversight.com/api/emailvalidation";
static string _apiToken = "00000xx0-00x0-00x0-00xx-x00xx000xxxx";
public static EmailValidationResponse ValidateEmail(EmailValidationRequest request, int timeoutInSeconds, Logger log)
{
EmailValidationResponse resps = new EmailValidationResponse();
using (var client = new HttpClient())
{
try
{
client.BaseAddress = new Uri(_apiURL);
client.DefaultRequestHeaders.Accept.Clear();
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
client.DefaultRequestHeaders.Add("ApiToken", _ApiToken);
client.Timeout = new TimeSpan(0, 0, 0, timeoutInSeconds);
HttpResponseMessage response = client.PostAsJsonAsync("", request).Result;
if (response.IsSuccessStatusCode)
{
resps = response.Content.ReadAsAsync<EmailValidationResponse>().Result;
}
else
{
// Incase of an error
log.Trace(response.ReasonPhrase);
}
}
catch (Exception ex)
{
log.Trace(ex);
}
}
return resps;
}